This is the 1st chapter in a multi-part series called “How to write a Python3 SDK library module for a JSON REST API in 15 simple steps“
Before going into battle, it’s important to do some reconnaissance. With any REST API, it’s important to get a feel for how the REST API behaves. Some REST APIs are written by professionals and are very easy to work with. And well, others are written by amateurs and are down-right terrible and inconsistent. Understanding how the API behaves before writing any code is the foundation for whether your wrapper library will be any good or not.
In this chapter, we’re going to cover:
- Installing Postman
- How we decided on an API
- Understanding the Documentation
- Authentication (Credentials, Tokens, Cookies, etc.)
- API versions
- Paths and Endpoints
- Data returned (JSON)
Installing Postman
Visit Google’s Postman website
Download and install per instructions depending on your desktop platform (Windows, Mac, or Linux)
How we decided on an API
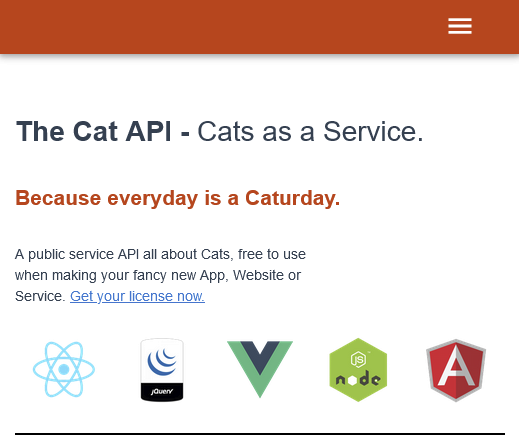
For this series, we will be using TheCatApi. It is a JSON REST API that yields images and info about cats. Because the Internet is full of cats, why not start here? (Actually, this documentation is very good and it seemed like a good idea to use an API with well-written documentation.)
Understanding the Documentation
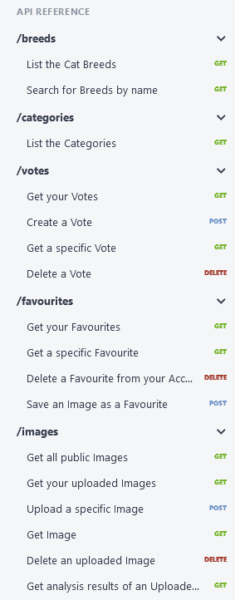
The documentation for TheCatApi can be found here: https://docs.thecatapi.com/
At the top is a link for “Authentication”, it reveals that:
- Authentication is done via API Auth key
(which you will need to register to get a free key.) - The most secure way to send your API Auth key is via the
x-api-key
header
(And not via the query parameter)
Down the left side of the docs, we can see multiple paths in the API Reference: breeds
, categories
, votes
, favourites
, images
.
Popping open each path, we can see that they use three different HTTP request types:
GET, POST, DELETE
As an example, we will click on the “Search & Pagination” link at the top:
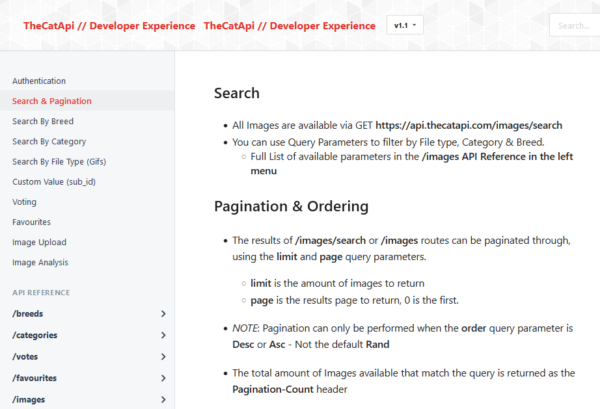
Here we can see that, to get a random image of a kitty, we will want to:
- put our API Auth Key in the
x-api-key
header - send a GET http request
- to https://api.thecatapi.com/v1/images/search
- and (maybe) use query parameters
limit
,page
, andorder
We can try this out by entering the information in Postman as shown in the image below. And then click SEND
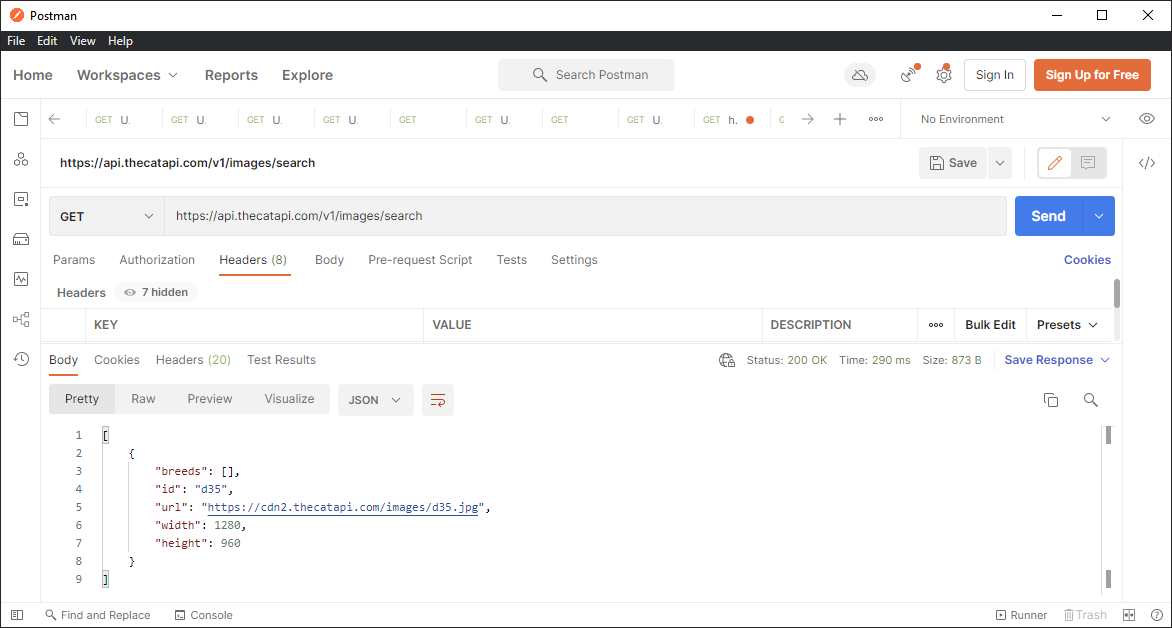
Authentication
Now that we have a handle on the basics of the documentation, we’re going to figure out Authentication. Some REST APIs will let you just hit their endpoints willy-nilly without giving any sort of credentials. But most websites these days require that you give some form of credentials. With TheCatApi, you can sign-up for free here and get your own API key: https://thecatapi.com/signup
Some websites rely on username / password combos. Some will give you an API Key that then generates a temporary time-limited cookie or a token which you must refresh once it expires. Other sites, like TheCatApi allow you to perform basic searches without any credentials, but more advanced features require that you sign-up and get an API Key.
We’ll show you how to implement such a system here.
API Versions
A well-implemented REST API will implement a version number. If done properly, whenever the REST API changes, the version number should increment. If ever the writer of the API makes “breaking changes” or significant improvements, the version number should increment.
When writing a library for a REST API, the code you write should be aware of version numbers and how the REST API behaves from version to version.
TheCatApi only has 1 version so far, but we’re going to account for this version anyway.
Data returned (JSON)
TheCatApi returns data in what has become the defacto industry standard: JavaScript Object Notation (aka. JSON)
In chapter 3, we will learn how to take JSON data and stuff the values into strong Python data models.
Paths and Endpoints
All good REST APIs have Paths and Endpoints that you can hit. Back in our Postman demo, we plugged in a path and endpoint: /images/search
. (images
is part of the path and search
is the endpoint, although frequently the entire “path & endpoint” together are referred to as just “the endpoint.”)
TheCatApi has 5 major paths: /breeds
, /categories
, /votes
, /favourites
, and /images
. Each path has its own set of endpoints (as found in the documentation) — A total of 17!
In chapter 4, we will try to implement some of the 17 paths/endpoints as methods that can easily be called and return strong data types that can be easily manipulated by your Python environment.
With all of this information about TheCatApi, we’re ready to start writing code!
One reply on “Step 1: Read the Docs and use PostMan to understand the REST API”
[…] Step 1: Read the Docs and use PostMan to understand the REST API […]